Implement A/B Testing with BlipHQ: A Developer's Guide to Data-Driven Decisions
07/09/2024
—
Admin
Use Cases
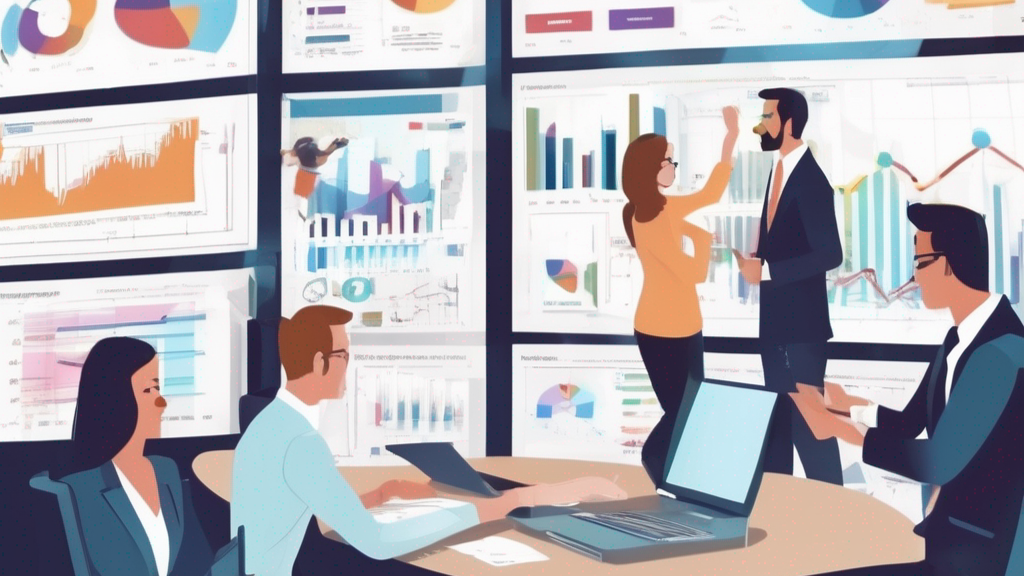
In this tutorial, we'll walk you through creating a server-side A/B testing system using BlipHQ and Python. We'll assume that necessary frontend functions for displaying variants already exist.
Step 1: Set Up BlipHQ
- Sign up for a BlipHQ account at https://bliphq.com
- Obtain your API key from the dashboard
- Set up your preferred notification channels (e.g., Telegram, Discord) and nickname it "abtestbot"
- Create a new project named "ABTestingProject"
Step 2: Implement A/B Test Variants
First, let's create a Python function to assign and retrieve variants:
import random
from flask import Flask, session, jsonify
app = Flask(__name__)
app.secret_key = 'your_secret_key' # Set this to a secure value
def get_variant():
variants = ['A', 'B']
if 'ab_test_variant' not in session:
session['ab_test_variant'] = random.choice(variants)
return session['ab_test_variant']
@app.route('/get_variant')
def variant_endpoint():
return jsonify({'variant': get_variant()})
Step 3: Apply Variant Changes
Now, let's create a function to determine what content to serve based on the variant:
def get_variant_content(variant):
if variant == 'A':
return {
'button_color': 'blue',
'headline': 'Welcome to our amazing service!'
}
else:
return {
'button_color': 'green',
'headline': 'Discover the power of our platform!'
}
@app.route('/get_content')
def content_endpoint():
variant = get_variant()
content = get_variant_content(variant)
return jsonify(content)
Step 4: Track User Interactions
Let's use BlipHQ to track user interactions with our variants:
import requests
BLIPHQ_API_KEY = "YOUR_API_KEY_HERE"
PROJECT_NAME = "ABTestingProject"
def track_event(event_name, variant):
url = "https://bliphq.com/api/push"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {BLIPHQ_API_KEY}"
}
payload = {
"message": f"A/B Test Event: {event_name}",
"project": PROJECT_NAME,
"nickname": "abtestbot",
"tags": ["abtest", event_name, variant]
}
requests.post(url, json=payload, headers=headers)
@app.route('/track_view')
def track_view():
variant = get_variant()
track_event('page_view', variant)
return '', 204
@app.route('/track_conversion', methods=['POST'])
def track_conversion():
variant = get_variant()
track_event('conversion', variant)
return '', 204
Step 5: Frontend Integration
Assuming the frontend exists, here's a simple example of how it might interact with our backend:
<script>
async function loadVariant() {
const response = await fetch('/get_content');
const content = await response.json();
document.getElementById('headline').textContent = content.headline;
document.getElementById('cta-button').style.backgroundColor = content.button_color;
// Track page view
fetch('/track_view');
}
function trackConversion() {
fetch('/track_conversion', { method: 'POST' });
}
// Load variant content when page loads
window.addEventListener('load', loadVariant);
// Attach conversion tracking to button click
document.getElementById('cta-button').addEventListener('click', trackConversion);
</script>