Build a Comprehensive Server Monitoring System with BlipHQ
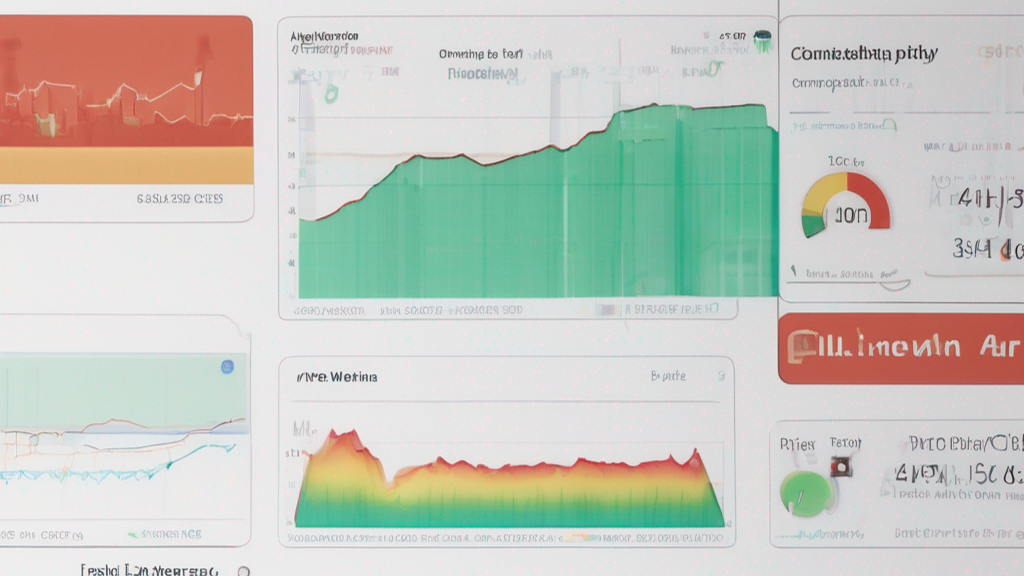
In this tutorial, we'll walk you through building a comprehensive server monitoring system using BlipHQ. You'll learn how to track various server metrics and receive real-time alerts for critical events.
Step 1: Set Up BlipHQ
- Sign up for a BlipHQ account at https://bliphq.com
- Obtain your API key from the dashboard
- Set up your preferred notification channels (e.g., Telegram, Discord) and nickname it "serveralert"
- Create a new project named "ServerMonitor"
Step 2: Implement Basic Server Monitoring
Let's create a Python script to monitor basic server metrics:
import psutil
import requests
import time
BLIPHQ_API_KEY = "YOUR_API_KEY_HERE"
PROJECT_NAME = "ServerMonitor"
def send_metric(metric_name, value):
url = "https://bliphq.com/api/push"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {BLIPHQ_API_KEY}"
}
payload = {
"message": f"{metric_name}: {value}",
"project": PROJECT_NAME,
"tags": ["server_metric", metric_name],
"data": {"value": value}
}
requests.post(url, json=payload, headers=headers)
def monitor_basic_metrics():
cpu_percent = psutil.cpu_percent()
memory_percent = psutil.virtual_memory().percent
disk_percent = psutil.disk_usage('/').percent
send_metric("cpu_usage", cpu_percent)
send_metric("memory_usage", memory_percent)
send_metric("disk_usage", disk_percent)
while True:
monitor_basic_metrics()
time.sleep(60) # Check every minute
Step 3: Monitor Server Requests
Let's add functionality to track server requests using a Flask middleware:
from flask import Flask, request
import time
app = Flask(__name__)
@app.before_request
def track_request():
request.start_time = time.time()
@app.after_request
def send_request_metric(response):
total_time = time.time() - request.start_time
send_metric("request_time", total_time)
return response
# Your Flask routes go here
Step 4: Monitor Processes and Containers
Now, let's add process and Docker container monitoring:
import docker
def monitor_processes():
for proc in psutil.process_iter(['pid', 'name', 'cpu_percent', 'memory_percent']):
send_metric(f"process_{proc.info['name']}", {
"cpu": proc.info['cpu_percent'],
"memory": proc.info['memory_percent']
})
def monitor_containers():
client = docker.from_env()
for container in client.containers.list():
stats = container.stats(stream=False)
cpu_usage = stats['cpu_stats']['cpu_usage']['total_usage']
memory_usage = stats['memory_stats']['usage']
send_metric(f"container_{container.name}", {
"cpu": cpu_usage,
"memory": memory_usage
})
while True:
monitor_basic_metrics()
monitor_processes()
monitor_containers()
time.sleep(60) # Check every minute
Step 5: Set Up Real-Time Alerts
Configure your BlipHQ account to send notifications for critical events. For example:
def check_critical_levels():
if psutil.cpu_percent() > 90:
send_alert("High CPU Usage", "CPU usage exceeds 90%")
if psutil.virtual_memory().percent > 95:
send_alert("Low Memory", "Available memory below 5%")
def send_alert(title, message):
url = "https://bliphq.com/api/push"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {BLIPHQ_API_KEY}"
}
payload = {
"message": f"ALERT: {title} - {message}",
"project": PROJECT_NAME,
"nickname": "serveralert",
"tags": ["alert", title.lower().replace(" ", "_")]
}
requests.post(url, json=payload, headers=headers)
Step 6: Analyze Server Performance
Regularly review your BlipHQ dashboard to gain insights into:
- Server resource utilization trends
- Request patterns and response times
- Process and container performance
- Frequency and types of critical alerts
Conclusion:
By following this tutorial, you've created a comprehensive server monitoring system using BlipHQ. You can now track various server metrics, receive real-time alerts, and access detailed analytics through the BlipHQ dashboard. This setup allows you to proactively manage your server infrastructure and quickly respond to potential issues.
Note:
Remember to secure your monitoring script and consider the performance impact of frequent monitoring. Adjust the monitoring frequency and metrics collected based on your specific needs and server capabilities.